Technology
May 21, 2014
How to Create Apps for Google Glass Using Mirror API With Spring Boot: Part 2 Logging In
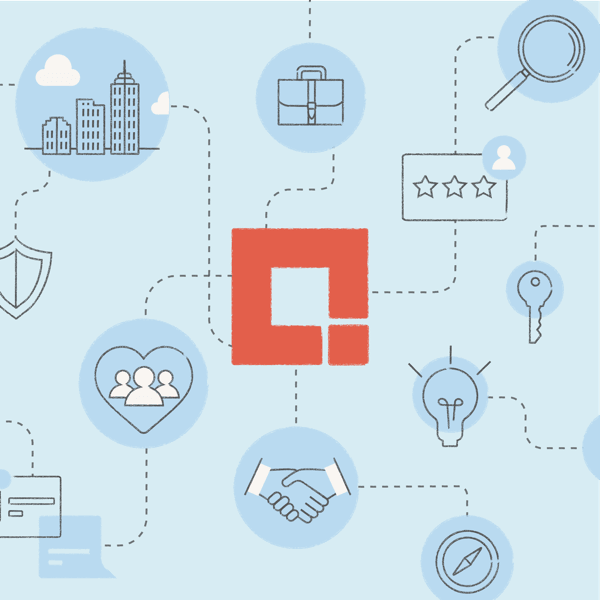
This three-part blog series is exploring how to create apps for Google Glass using Mirror API with Spring Boot. Last time we looked at the Spring Boot configuration and today we’re going to dive into the login process.
Logging in With Google – Client ID and Secret
The first step in allowing users to log in to the webserver using their Google account is to register the server with Google’s developer console. Google will generate a pair of unique “client ID” and “client secret” codes for each registered server to use with all of its users’ OAuth 2.0 logins.
Google’s Mirror API quickstart contains instructions on how to generate a client ID and secret for a web server. However, those instructions are slightly outdated since Google has recently updated their developer console. The new steps are as follows:
1. Go to https://code.google.com/apis/console/
2. Click on “Credentials” under “APIs & auth”
3. Click “Create New Client ID”
4. Set the application type to “web application”
5. Leave the “Authorized Javascript Origins” blank
6. Set the “Authorized Redirect URI” to http://localhost:8080/spring-boot-glass/oauth2callback. This value must reflect where the server is being hosted from. This URL assumes that the server is running locally on port 8080 with “spring-boot-glass” as its context root. This value will need to be changed if the webserver is not being accessed locally.
7. Record the client ID and client secret for later use when configuring the local server
The client ID and secret are loaded into the Spring Boot Glass example as Java properties in OAuthResources.java using Spring’s @Value annotation. Since the client ID and secret should not be committed in source control, an easy way to securely add them to the project is to set them as VM arguments.
To start Spring Boot Glass in a webserver in Eclipse with these arguments, perform the following steps:
1. Create a new Tomcat 7 server in the Eclipse Server tab
2. Add the Spring Boot Glass project to it
3. Double click on the new server, go to “open launch configuration,” and add the following two VM arguments:
-Doauth.google.clientid=”client_id_from_google”
-Doauth.google.clientsecret=”client_secret_from_google”
If the server fails to boot or an error such as “invalid_client” occurs when going to http://localhost:8080/spring-boot-glass/, then either the values of the VM arguments are incorrect, or the server’s port or project’s context root may need to be changed to match the “Authorized Redirect URI” provided while generating the client ID and secret on Google’s API console. The port can be changed by double clicking the server name in the Server tab and editing its properties, and the context root can be changed by going into the project’s properties within the Web Project Settings section.
Logging in with Google – OAuth 2.0 Redirect Dance
To allow users to log in using their Google account, the server must redirect the user to Google, which will then redirect the user back to the server. These steps are handled by OauthController.java, which was reconstructed from Google’s Mirror API quickstart by extracting code embedded within their login JSP and putting it into a reusable Spring controller. It makes heavy use of AuthUtil.java, which is a stripped down version of the AuthUtil.java found in Google’s Mirror API quickstart that has been upgraded to remove unused functions and the use of deprecated Google APIs.
The steps for the OAuth 2.0 redirect “dance” in OauthController.java are as follows:
1. The user goes to the server’s OAuth login page. They can either directly go to http://localhost:8080/spring-boot-glass/oauth2callback or they can go to any other page (such as the main page at http://localhost:8080/spring-boot-glass) and OauthInterceptor.java will automatically redirect them to the login page if they are not logged in yet.
2. The page redirects the user to Google’s OAuth 2.0 login URL with the following information (as defined in OAuthResources.java):
a. Server’s client ID
b. Server’s client secret
c. Permissions for the server to access Google resources on behalf of the user, such as their Glass device.
d. URL to redirect the user back to after they log in with Google (passed from the currentControllerUrl String in OauthController.java).
3. The user logs in with Google on their servers.
4. The user accepts the original’s server’s requests to access their Google resources.
5. Google redirects the user back to the server with a code to access the user’s information.
6. The server makes one last request to Google using the code to get the user’s ID and OAuth 2.0 access token and refresh token. This information is stored in a Credential object from Google’s OAuth 2.0 libraries that all of their Mirror library calls utilize, allowing the developer to not have to worry about using the access or refresh tokens directly.
Once the user is logged in, OauthController stores the user’s ID inside a session scoped bean, OAuthSession.java. This bean can be injected into any other Spring component and used in conjunction with AuthUtil to retrieve the user’s Credential object during their session. Since OAuthInterceptor.java always redirects the user to the login page if the user’s session does not have a user ID, it is safe to assume that the session scoped user ID and Credential variables are always available for Mirror API calls in the rest of the server’s Spring components.
If the user ever revokes Google permissions while they are still logged in to the Spring Boot Glass server, a Spring MVC global exception handler, ControllerExceptionHandler.java, will redirect them back to the login page again. No configuration files are required to make this exception handler work; its @ControllerAdvice annotation allows it to be automatically registered as a global exception handler for all Spring MVC Controllers.
Note that during step two, OauthController.java sets “approval_prompt” to “auto” on the Google redirect URL. This forces Google to only ask for the user’s permission to allow the server to access their resources once (as seen in step four). After the user has logged in to the server for the first time, they will never be prompted with the permission screen again. They can just log in with Google to allow the server to make requests while their session is alive without needing to persist any of their information. As an added bonus, if the user has recently logged into Google, they will actually automatically log in to the server without seeing any prompts at all after their initial visit. This allows the Spring Boot Glass example to provide seamless user login functionality without requiring a database.
For more detailed information on how Google’s OAuth 2.0 APIs work, see Google’s documentation. Knowing the inner-workings of OAuth 2.0 is not necessary to use Google’s APIs, but it can help debug implementation issues.
If you have any questions about the Spring Boot Glass project, please feel free to use the comments section below or contact us at info@credera.com. You can also follow us on Twitter at @CrederaOpen and connect with us on LinkedIn for additional Open Technologies insights.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings