Technology
May 14, 2014
How to Create Apps for Google Glass Using the Mirror API With Spring Boot – Part 1
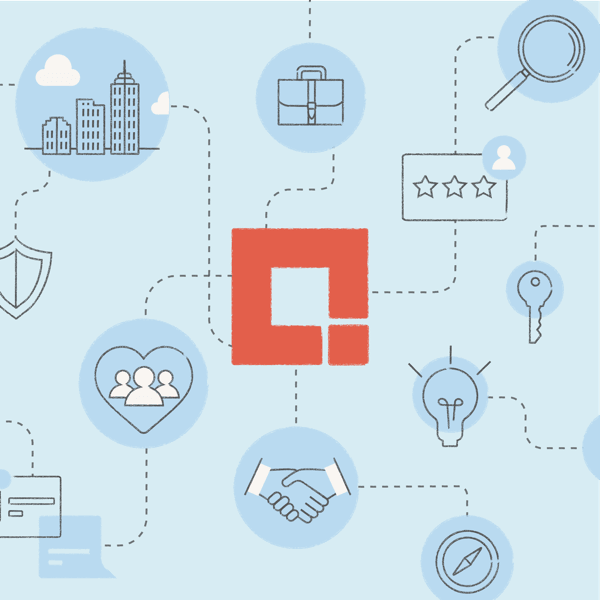
Now that Google Glass has become available for public purchase in the US, you may have access to a Glass device and be interested in making an application for it. The easiest way to do this is with Google’s Mirror API, which is a new way to write apps that actually run on webservers instead of the device itself. It also doesn’t require any prior Android programming experience. This three-part blog series will explore how to get started with the Google Glass Mirror API using a Java project built on Spring Boot.
Google has provided two ways to make apps for Glass – using an APK running Android code on the device itself, or using the Mirror API. The Mirror API allows a webserver to access a user’s Glass device by interacting with it as a RESTful resource. Once a user gives a webserver permission to access their Glass via OAuth 2.0, the webserver can push and pull content to and from the device without having to install any new software on it. While certain types of complex applications that heavily utilize Glass’s sensors (such as games or real-time image processing applications) will require native code running on the device, the Mirror API can easily handle applications that deal with simple text and media displays and transfers.
Google provides Java libraries that abstract the lower level details of the Mirror API’s OAuth 2.0 authentication and HTTP rest calls away from the developer. Thus, using the Mirror API in Java doesn’t require detailed knowledge of how to implement OAuth 2.0 or how to make REST API calls at all – it merely requires interacting with Google’s existing client API library. If you have implemented OAuth 2.0 on other projects (perhaps using libraries such as Spring Security’s OAuthRestTemplate) or made RESTful web services yourself before (perhaps with Spring MVC’s RestControllers), none of those technologies are required to access the Mirror API itself since Google’s libraries handle those calls.
Mirror API Quickstart Projects
Google provides a sample Java Mirror API quickstart application. However, their example utilizes the servlet API and JSPs for its content, uses deprecated methods from Google’s own libraries, and hasn’t been updated in almost a year (as of the time of this writing). Combined, these attributes make it a little difficult to use as a basis for easily creating a new Glass application built on a modern Java framework.
The following sections detail a custom, improved version of Google’s sample Java Mirror API application called Spring Boot Glass, hosted on GitHub. This example shows how to get started with the Glass Mirror API using the Spring framework. It makes use of Spring’s dependency injection annotations, Spring MVC, and Thymeleaf to provide a simple website where users can push custom text content to their Glass devices. To gain the advantages of Spring without dealing with boilerplate default configuration files, it is built on Spring Boot using Gradle for dependency management.
Spring Boot Configuration
When people think of the Spring framework, they tend to think of two things: access to libraries that greatly simplify web development and lots of XML configuration. Spring Boot can be used with specially annotated Java classes to completely remove the XML configuration. Instead, a developer can configure what components their program has access to via Gradle or Maven imports of popular Java libraries or pre-existing Spring Boot “starter” projects. This makes it easy to add new functionality such as Spring Security, Spring Data, and Thymeleaf, without requiring large amounts of configuration.
For example, to gain access to Spring Security, a developer can add a Spring Security starter project to their build.gradle or Maven POM file, which will put appropriate versions of all the Spring Security JARs on the classpath and automatically assign default values to all beans that would normally have had to be explicitly declared in the configuration XML. While the Spring Boot Glass project does not use Spring Security itself, it can be added by modifying the project’s build.gradle file and performing the steps in Spring’s documentation.
The Spring Boot Glass project’s dependencies are managed within its build.gradle file. To use this file to automatically download all libraries required to use the project in Eclipse, install the Gradle Integration for Eclipse plugin through the Eclipse Marketplace in the Help menu, import the project as a Gradle project, right click the project, and perform a Gradle Refresh All operation.
Some configuration is required however, even with Spring Boot. This can be done in a series of specially annotated configuration classes. Spring Boot Glass uses three classes to satisfy this requirement.
1. Main.java is responsible for starting Spring Boot.
– By using @Configuration this class acts as a replacement for the main Spring configuration XML file.
– Its @EnableAutoConfiguration annotation sets up default configurations for all applicable packages detected on the classpath.
– The @ComponentScan annotation automatically makes all classes that use @Component, @Service, or @Repository in packages lower than it eligible for dependency injection. Be sure to place this class high in the package hierarchy, since it cannot automatically locate classes in higher packages. This is why Main.java is located within the org.jdw package in the Spring Boot Glass project, while all other project code is in one of org.jdw’s child packages.
2. MvcConfig.java uses @Configuration to add one more component that would normally be placed in a Spring MVC configuration XML file. It defines a bean to use for HTTP request interceptions, which is used to make all requests pass through a custom OauthInterceptor.java component to ensure that the user has logged in with their Google account before they can access any page that isn’t the login page.
3. MainWebXml.java removes the need to create a web.xml file to deploy the application on a Tomcat server. It doesn’t need any special annotations, it just needs to extend SpringBootServletInitializer and provide a reference to Main.class. Spring boot will automatically detect this configuration class on the classpath without any other resources specifying its location.
If you have any questions about the Spring Boot Glass project, please feel free to use the comments section below or contact us at info@credera.com. You can also follow us on Twitter at @CrederaOpen and connect with us on LinkedIn for additional Open Technologies insights.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings