Technology
Sep 11, 2009
Creating a single-click Windows Mobile 6.0 application installer exe
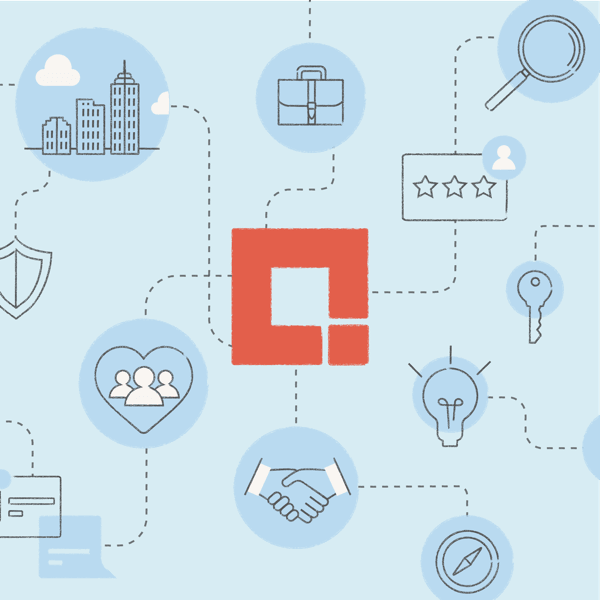
Anyone who has ever installed an application onto a Windows Mobile device has gone through the process of downloading and installing a CAB file. Unfortunately, this process can be a bit tedious and leaves ample room for derivation from the proper technique. At this point, it begs the question, why can’t there by a single setup.exe file that will install the CAB, clean up after itself, and copy files to the system, similar to the way a desktop application works?
Unfortunately, Microsoft does not have a solid solution or tool that accomplishes this in a highly customizable fashion. Therefore, I’ve created a blueprint solution that has an *.exe output to accomplish this task.
These are the key steps to creating this solution:
Create a Windows Mobile Device application
Add the CAB file and any other files to the project resources
Configure the file locations on the mobile device
Invoke the installation of the CAB
Clean up the unnecessary files on the device
Create a Windows Mobile Forms application
Create a new solution in Visual Studio. Add a new Project of type Smart Device –> Device Application. All of our code logic will reside in the Form1.cs class. This is not a restriction, as the code can live in a separate class.
Add the CAB file and any other files to the project resources
Drag and drop the CAB file and any other files necessary (icons, text files, “readme” files, etc) to the project resources folder. It is important that these files live in this folder, as we will be using the application resources object model to extract and use these files.
Configure the file locations on the mobile device
Before we can actually use the CAB file to install our application, we need to copy it to the device. We will use a simple file stream to copy the file , and the unmanaged function SHGetSpecialFolderPath to configure the location. We will PInvoke this method to expose it to our managed code. The method takes IntPtr values, and each value corresponds to a particular folder location. In this case, we will copy our files to the Program Files location. It is important to use this instead of hard coding a path, so that it will work for any Windows Mobile device whether or not the user has altered the default file system configuration.
Here is the code snippet:
[code lang=”csharp”]const int CSIDL_PROGRAM_FILES = 0x0026; //The program files folder.
[DllImport(“coredll.dll”)]
static extern int SHGetSpecialFolderPath(IntPtr hwndOwner, StringBuilder lpszPath, int nFolder, int fCreate);
private string getPath(int folderCSIDL)
{
StringBuilder resultPath = new StringBuilder(255);
SHGetSpecialFolderPath((IntPtr)0, resultPath, folderCSIDL, 0);
return resultPath.ToString();
}
void CopyCab()
{
string cabPath = getPath(CSIDL_PROGRAM_FILES) + “\\Application.CAB”;
// COPY CAB FILE
FileStream writer = null;
try
{
writer = new FileStream(cabPath, FileMode.Create);
writer.Write(Properties.Resources.CAB, 0, Properties.Resources.CAB.Length);
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
finally
{
if (writer != null)
{
writer.Flush();
writer.Close();
}
writer = null;
}[/code]
This technique can be applied to any file that needs to be on the file system. A good example of a file that a developer might want to access before the actual unpacking of the CAB file is a Disclaimer text file that can be used to display information prior to installation.
Invoke the installation of the CAB
Now that we have our CAB file on the device, in a location that we configured, we can install it. This is a quite simple step, and can be accomplished using the Process.Start() method. We will use the WaitForExit() method to block our code from running until the installation is complete. Remember our cabPath variable we declared earlier; we will use this path to kick off the installation.
[code lang=”csharp”]Process installProc = null;
try
{
installProc = Process.Start(cabPath, null);
installProc.WaitForExit();
}
catch (Exception ex)
{
MessageBox.Show(ex.Message);
}
[/code]
Clean up the unnecessary files on the device
Now that our application has been installed, we want to do a little clean up to erase any footprints we made during our custom installation. Using the System.IO assembly and the File.Delete() method, we can accomplish this.
[code lang=”csharp”]try
{
this.Close();
File.Delete(cabPath);
}
catch
{
MessageBox.Show(“Installer could not clean up files. Please delete them manually.”);
}
[/code]
If any other files were added, like a disclaimer, they can be deleted in the same way.
The output for this project will be in the form of an exe file. When a user runs the exe, the installation process is smooth and requires minimal effort or thought on the part of the user.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings