Technology
May 28, 2014
How to Create Apps for Google Glass Using Mirror API With Spring Boot: Part 3 Making Mirror API Calls
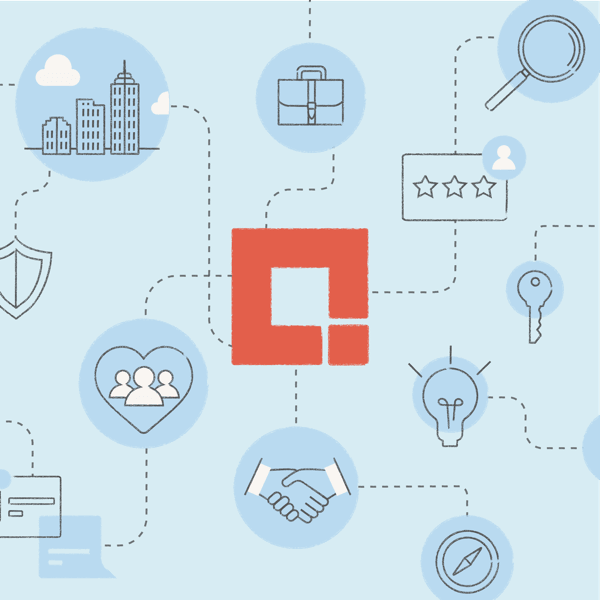
This three-part blog series is exploring how to create apps for Google Glass using Mirror API with Spring Boot. Last time we looked at the login process and today we’re going to explore how to make mirror API calls.
The following class diagram details the flow that will be used to make a Mirror API call.
Spring Boot Class Diagram
Google’s Mirror library requires a user’s Credential object for all of its Mirror API calls. Now that the user has logged in, their Credential object can easily be accessed from any Spring component. For example, Spring Boot Glass contains a service called TimelineService.java that uses a method called “credential()” to access the current session scoped user ID and its corresponding Credential object. This code can be reused in any Spring component to access the appropriate session Credential object for Mirror API calls.
@Autowired
private AuthUtil authUtil;
@Autowired
private OAuthSession session;
/**
* @return The OAuth {@link Credential} for the current user.
*/
private Credential credential() throws IOException {
String currentUserId = session.getUserId();
Credential credential = authUtil.getCredential(currentUserId);
return credential;
}
TimelineServie makes its Mirror API calls though MirrorClient.java, which is a facade that makes it easier to use Google’s Mirror libraries. MirrorClient was adapted from Google’s Java Mirror API quickstart with minor modifications to add additional functions. It uses Google’s static com.google.api.services.Mirror object to make HTTP calls to Google’s Mirror API endpoints, requiring the current user’s Credentials object as an argument within each call. The Mirror object abstracts away all knowledge of HTTP endpoint URLs and JSON serialization, offering an easy way to interact with the Mirror REST API through builder-pattern syntax. MirrorClient.java is a great central location for adding new abstractions over Mirror library methods to make it easy for other services to reuse them.
Google Glass displays information to users in the form of “cards” that form up a “timeline” the user can navigate through. TimelineService uses MirrorClient’s insertTimelineItem method to insert a new static card on the Glass user’s timeline with user-specified text. When the user taps the card, an option to delete it will appear, as specified by the menu item added to the TimelineItem.
TimelineItem timelineItem = new TimelineItem();
timelineItem.setText(text);
MirrorClient.addDeleteMenuItem(timelineItem);
MirrorClient.insertTimelineItem(credential(), timelineItem);
TimelineService.insertTimelineItem
Processing User Input
The text placed on the TimelineItem comes from a form in index.html, a Thymeleaf HTML page. The form submits the user’s text to a Spring MVC controller at IndexController.java, which serializes the form into a Message.java object. By using the @Valid annotation in the controller, the JSR303 bean validation annotations on the Message argument’s fields are automatically evaluated and applied to the BindingResult argument if any violations have occurred. The model argument is used to insert data within the returned index view.
public String submitText(Model model, @Valid Message message, BindingResult result)
IndexController.java form input method arguments
@NotBlank
@Size(max = MAX_TEXT_LENGTH)
private String text;
Message.java JSR303 Validation Annotations
The JSR303 @Notblank and @Size annotations allow the controller to reject the form input if the BindingResult argument contains errors. This allows sanitized user input to be safely brought through the controller layer to the service layer. While using Spring MVC and validation annotations steps are not necessary to use the Mirror API, they serve as an example of one of the advantages of using the Spring framework.
Next Steps
Pushing text to a static timeline card is just one of many Mirror API capabilities. You can download the Spring Boot Glass project and make your own new methods in TimelineService.java that access MirrorClient.java to try out other Mirror API methods on your own Glass device today. MirrorClient can be extended with more methods that use Google’s static com.google.api.services.Mirror object to add more functionality beyond the Spring Boot Glass starter project’s offerings. See Google’s Java Mirror API documentation for information on more Glass interactions that can be added.
The Spring Boot Glass project could be expanded beyond additional Mirror API calls by adding Spring Data to persist user accounts and Spring Security to grant different users access to different features and pages.
Apps that require Glass access outside the life of a single session may have to persist the user’s refresh token to reconstruct a Credential object for that user when they are not actively using the website. Some Glass application examples include timed reminders, notifications when certain events occur (e.g., bad traffic conditions on a user’s route home), or an application that pushes a daily photo.
The Spring Boot Glass example does not cover persisting user information for long-term access. Google provides credential store documentation on how to re-create a user’s Credential object outside of an HTTP session. The MemoryDataStoreFactory in AuthUtil.java can be replaced to use different types of CredentialStores other than the MemoryStore to persist this information. Alternatively, a Credential object can be re-created without changing the credential store by persisting the user’s refresh token and making manual OAuth 2.0 calls to retrieve new access tokens at a later date, as described by the calls documented in Google’s webserver OAuth documentation. See Google’s Credential JavaDocs for additional information on how to construct a Credential object using a refresh token.
By using Spring Data, Spring Security, and one of these alternate methods of reconstructing a Credential object using a persisted refresh token, it would also be possible to let users create an account on your website that only needs to be linked to Google once, instead of using Google for every login. While most standalone Mirror API applications do not require separate user accounts, some applications that add Mirror API functionality to existing services (such as Facebook) need to make use of this one-time account linking approach.
If you have any questions about the Spring Boot Glass project, please feel free to use the comments section below or contact us at info@credera.com. You can also follow us on Twitter at @CrederaOpen and connect with us on LinkedIn for additional Open Technologies insights.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings