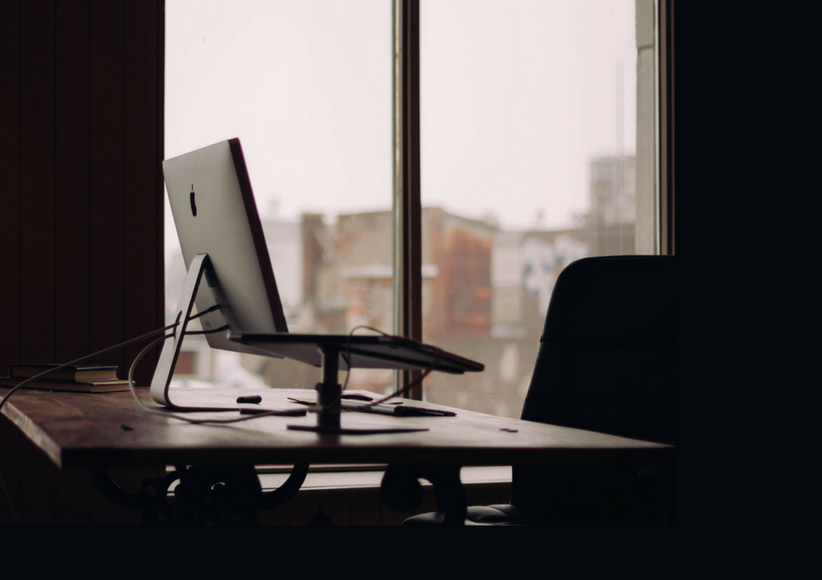
Microsoft Azure Application Gateway is a Layer 7 application delivery controller (ADC) offered as a service in Azure. It provides load balancing, SSL termination, end-to-end SSL, URL path-based routing, and basic web application firewall (WAF) functionality. In a previous blog post, I addressed the question “Is Azure Application Gateway a Good Fit for My Application?” This blog post assumes you’ve already decided an Azure Application Gateway is the way you want to go, but now you need to start configuring it. While you can use the Azure Portal to configure most elements of the Azure Application Gateway, to do some tasks you need to use PowerShell. And the good news is that once you understand how the Azure Application Gateway PowerShell works, it is much easier and faster than using the Portal to add configurations.
Creating a New Gateway vs. Working With an Existing Gateway
Microsoft does a decent job outlining how to set up a brand-new gateway with PowerShell for various scenarios in their documentation here. As you look through instructions for say, “Create an application gateway for hosting multiple web applications,” the effort to set up all the variables and components probably seems more complicated than just using the Portal to create a new gateway. I’ve found that it is easiest to create a new Application Gateways in the Portal but to update gateway configurations in PowerShell.
Whether you create your gateway in the Azure Portal or PowerShell, there will likely come a time when you want to add a new configuration for a new site or set of back-end resources. This is where PowerShell comes in handy, especially if you take the time to write a reusable script to update all the configuration items based on your needs. For this blog post, we will just look at running commands in an interactive fashion. My preferred method of setting up these configuration changes is to lay them out in the ISE Script Pane and then run them with the Run-Selection option. Also, it should be noted that this blog post uses the AzureRm PowerShell commands version 4.4.0.
Working With Application Gateways in PowerShell
First, let’s cover the convention for working with Application Gateways in PowerShell. The first step for updating any existing Gateway is to load the whole gateway configuration from Azure into a PowerShell object.
$AppGw = Get-AzureRmApplicationGateway -Name MyAppGw -ResourceGroupName MyAppGwRG
From here, all the changes we make are to the PowerShell object “$AppGw” until we are ready to commit the gateway back to Azure with the following command:
Set-AzureRmApplicationGateway -ApplicationGateway $AppGw
Between those two commands we can add and/or remove any configuration components. While the Set-AzureRmApplicationGateway command still takes a while to complete, you only need to run it once for any number of configuration component changes—unlike making those changes in the Portal, which will resave the gateway for each component that is updated.
Add a Multi-Site End-to-End SLL Configuration
As an example of adding a complex configuration, let’s look at the commands we will run to set up a multi-site listener with end-to-end SSL. First, remember to load the gateway into a PowerShell object, then we can start adding the elements of our configuration. I’ll start by loading the public SSL certificate to the gateway. The public certificate should be in PFX format with a password. So after you get it back from your certificate authority, load it into Certificate Manager on a Windows machine and re-export it with the private key and a password to the PFX format. Once you have the PFX file, add it to the gateway PowerShell object with the following command:
Add-AzureRmApplicationGatewaySslCertificate -ApplicationGateway $AppGw -Name "FrontendCertName" -CertificateFile "C:\Path\To\File\url.myapp.com.pfx" -Password "Pass@word1"
$AGFECert = Get-AzureRmApplicationGatewaySslCertificate -ApplicationGateway $AppGW -Name "MyAppCert"
If you don’t already have port 443 set up on the gateway, we can add it now. If you already have port 443 set up, we just need to save it to a PowerShell object.
Add-AzureRmApplicationGatewayFrontendPort -ApplicationGateway $AppGw -Name "appGatewayFrontendPort443" -Port “443”
$AGFEPort = Get-AzureRmApplicationGatewayFrontendPort -ApplicationGateway $AppGw -Name "appGatewayFrontendPort443"
Now that we have the front-end SSL certificate and port saved to PowerShell objects, we need to save the front-end IP configuration to an object, then create the front-end listener using these objects, and then save the listener in its own object.
$AGFEIPConfig = Get-AzureRmApplicationGatewayFrontendIPConfig -ApplicationGateway $AppGw -Name "appGatewayFrontendIP"
Add-AzureRmApplicationGatewayHttpListener -ApplicationGateway $AppGW -Name "MyAppListener" -Protocol Https -FrontendIPConfiguration $AGFEIPConfig -FrontendPort $AGFEPort -HostName "url.myapp.com" -RequireServerNameIndication true -SslCertificate $AGFECert
$AGListener = Get-AzureRmApplicationGatewayHttpListener -ApplicationGateway $AppGW -Name "MyAppListener"
Next let’s create the back-end pool for our web servers and save that back-end pool into its own PowerShell object. Note: I’m using IP addresses here, but you can also use fully qualified domain names.
Add-AzureRmApplicationGatewayBackendAddressPool -ApplicationGateway $AppGW -Name "AGMyAppBEP" -BackendIPAddresses "10.10.0.24", “10.10.0.25”
$AGBEP = Get-AzureRmApplicationGatewayBackendAddressPool -ApplicationGateway $AppGW -Name "AGMyAppBEP"
The Azure Application Gateway requires an authentication certificate for the back-end server when implementing end-to-end encryption so that the gateway will only forward traffic to a back-end server if it has the expected SSL certificate. For our setup on the gateway, we need to upload the public certificate that the back-end servers are using and save it into its own PowerShell object.
Add-AzureRmApplicationGatewayAuthenticationCertificate -ApplicationGateway $AppGW -Name "MyAppPublicCert" -CertificateFile "C:\Path\To\File\internalurl.myapp.com.cer"
$AGBECert = Get-AzureRmApplicationGatewayAuthenticationCertificate -ApplicationGateway $AppGW -Name "MyAppPublicCert"
The next element to configure is the back-end HTTP settings, which configures HTTPS over 443 and sets the authentication certificate that the gateway will look for on the back-end servers.
Add-AzureRmApplicationGatewayBackendHttpSettings -ApplicationGateway $AppGW -Name "AGMyAppHTTP" -Port 443 -Protocol Https -CookieBasedAffinity Enabled -AuthenticationCertificates $AGBECert
$AGHTTP = Get-AzureRmApplicationGatewayBackendHttpSettings -ApplicationGateway $AppGW -Name "AGMyAppHTTP"
Finally, we tie all the PowerShell objects we’ve created together with the routing rule.
Add-AzureRmApplicationGatewayRequestRoutingRule -ApplicationGateway $AppGW -Name "AGMyAppRule1" -RuleType basic -BackendHttpSettings $AGHTTP -HttpListener $AGListener -BackendAddressPool $AGBEP
Now that we’ve added all the configurations for the app to the Application Gateway PowerShell object, we just need to apply them to the gateway.
Set-AzureRmApplicationGateway -ApplicationGateway $AppGw
Removing Configuration Components
If you’ve worked with the Application Gateway in the Azure Portal you may have noticed that there isn’t a way to delete components, but we can do it in PowerShell. For an example, the following will remove the configuration we just created for my app above:
$AppGw = Get-AzureRmApplicationGateway -Name MyAppGw -ResourceGroupName MyAppGwRG
Remove-AzureRmApplicationGatewayRequestRoutingRule -ApplicationGateway $AppGw -Name "AGMyAppRule1"
Remove-AzureRmApplicationGatewayBackendHttpSettings -ApplicationGateway $AppGw -Name "AGMyAppBEP "
Remove-AzureRmApplicationGatewayAuthenticationCertificate -ApplicationGateway $AppGw -Name "MyAppPublicCert"
Remove-AzureRmApplicationGatewayHttpListener -ApplicationGateway $AppGw -Name "MyAppListener"
Remove-AzureRmApplicationGatewaySslCertificate -ApplicationGateway $AppGw -Name "MyAppCert"
Remove-AzureRmApplicationGatewayBackendAddressPool -ApplicationGateway $AppGw -Name "AGMyAppBEP"
Set-AzureRmApplicationGateway -ApplicationGateway $AppGw
The one thing to remember is that you can’t remove a component if it has an existing dependency, so for example, you can’t remove a listener, HTTP settings, or back-end pool if there is a routing rule that is using it.
The full documentation for Azure Application Gateway PowerShell version 4.4.0 can be found here. Have questions about configuring your Azure infrastructure? Credera has extensive experience in cloud infrastructure design and implementation. We would love to discuss potential cloud and infrastructure solutions with you—contact us at findoutmore@credera.com.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings