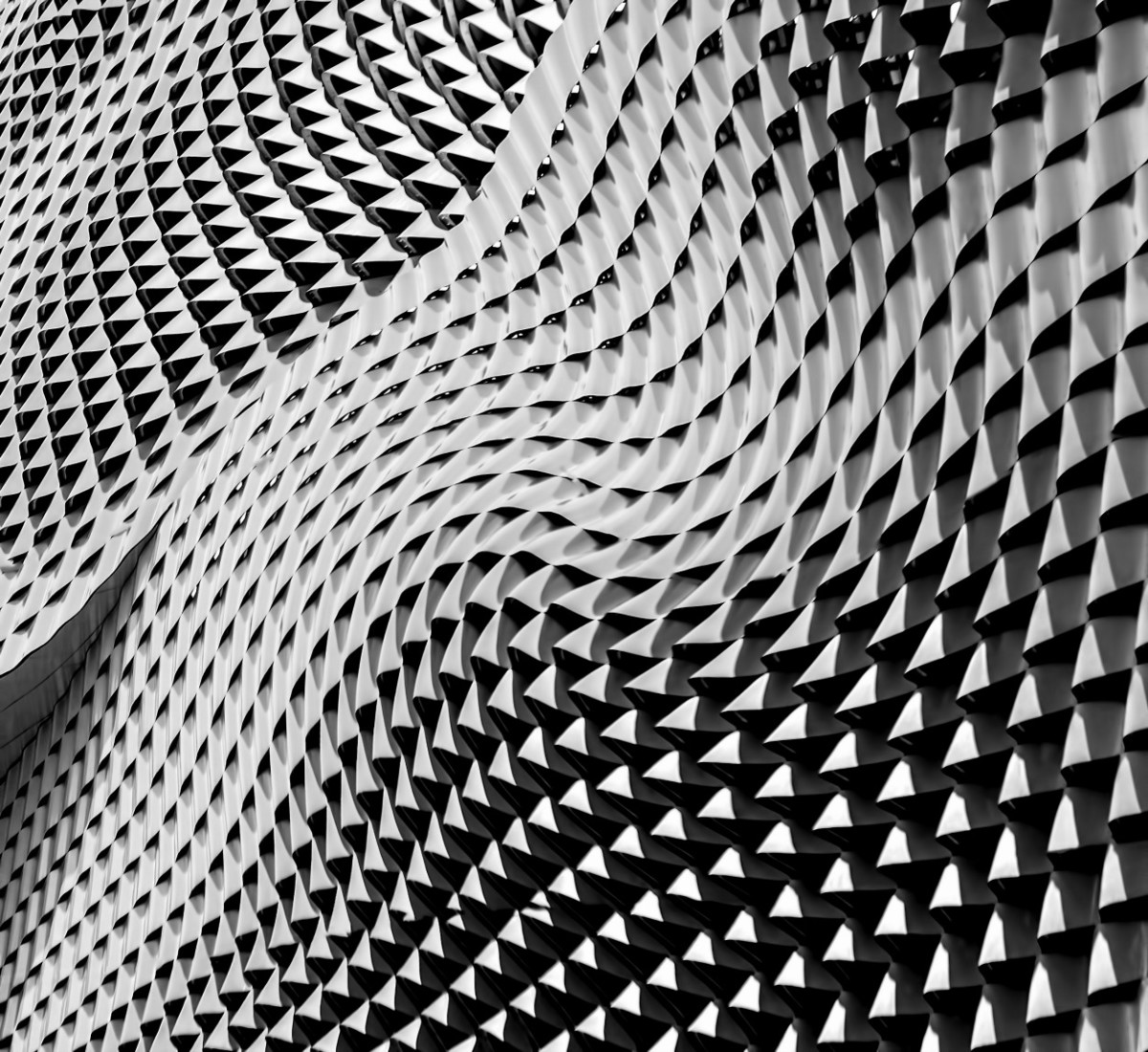
The purpose of this blog post is to give a brief overview of the circuit breaker pattern, where it can be used, and show a few examples of the excellent support for this pattern in Spring Boot provided by Netflix’s Hystrix library.
The concept of the circuit breaker pattern is borrowed from the field of electronics. If you’ve ever had to reset the electric breaker panel switches in your house after an electrical storm or power surge, you are already familiar with the concept. The circuit breaker is meant to protect a system from total failure by stopping the propagation of electricity that can overload the system or cause further damage to other subsystems.
Since modern software applications have the same need for fault tolerance and resiliency, we apply this concept by replacing electricity with data. This pattern is crucial in applications that use or rely on data and connectivity to external services (local network or cloud based), protecting the application from total failure when any external service goes down. The pattern is also used to make your application a good steward of the resources it depends on. For example, temporarily halting requests to a service that is busy can be beneficial as it lessens the burden placed on it and gives that external system time to recover.
A good implementation of the circuit breaker pattern allows for fine-grain control of how and when the circuit will trip and when to automatically close the circuit again. It will also provide mechanisms to return some default data or other methods of graceful degradation. Fortunately the open source library Hystrix from Netflix provides all of this and more, and Spring Boot makes it super easy to integrate using the ‘Spring Cloud Netflix’ package.
There are other excellent guides available that walk through the process of setting up the circuit breaker with Spring Boot (see Resources section below). Like most Spring Boot integrations, it is relatively straightforward. With these three steps, you should be off to a good start.
Add the springframework.cloud:spring-cloud-starter-hystrix dependency to your build file.
Add the @EnableCircuitBreaker annotation to your main application class (typically the class with the @SpringBootApplication annotation on it).
Annotate with @HystrixCommand the methods in your @Service or @Component classes that you wish to protect.
Here is an example of a simple class using the circuit breaker pattern with the fallback mechanism:
@Service
public class PingService {
private OkHttpClient okHttpClient;
public PingService() {
okHttpClient = new OkHttpClient();
}
@HystrixCommand(fallbackMethod = “pingFallback “)
public String pingGoogle() throws IOException {
final Request request = new Request.Builder()
.url(“https://google.com/”)
.get()
.addHeader(“cache-control”, “no-cache”)
.build();
okHttpClient.newCall(request).execute();
return “Ping to Google was successful!”;
}
private String pingFallback() {
return “Resource is busy or offline”;
}
}
In this simple example, Hystrix will keep track of how many IOExceptions occur within a rolling time window. Once an error threshold is crossed all requests to the method will be forwarded to the method defined in the ‘fallbackMethod’, then it resumes calling the original method after the sleep window duration has passed. In other words, calling the ‘pingGoogle’ method will return the output (“Ping to Google was successful!”). If Google was down for some reason or the application using this service loses connectivity, calls to ‘pingGoogle’ will temporarily return the output of the ‘pingFallback’ method (“Resource is busy or offline”) until Google is back up or connectivity is restored.
Hystrix’s default and custom Error Threshold %, Sleep Window duration and other settings (see below in Resources section) can be configured in the application.yml file:
hystrix:
command:
default:
circuitBreaker:
errorThresholdPercentage: 50 # 50%
sleepWindowInMilliseconds: 5000 # 5s
customCommandKey:
fallback:
enabled: false
circuitBreaker:
errorThresholdPercentage: 75 # 75%
sleepWindowInMilliseconds: 15000 # 15s
The ‘customCommandKey’ overrides the ‘default’ settings and can be applied to individual methods or classes using the ‘commandKey’ property of the @HystrixCommand. It is important to note that these custom named command keys must be unique since they are shared by the whole application and are used by Hystrix for tracking circuit breaker states, caches, and reporting. For example:
@HystrixCommand(fallbackMethod = “pingFallback”, commandKey = “customCommandKey”)
public String pingGoogle() throws IOException {
In this example, the method will have different Error Threshold % and Sleep Window duration than the default settings, but also note that even though a ‘fallbackMethod’ is defined, the configuration for this ‘customCommandKey’ has ‘fallback’ disabled. This shows the flexibility of the fine-grain control that can be useful in certain scenarios for changing behavior of the code without re-compiling or re-deploying the application.
If you are interested in learning more, check out the list of resources below and leave a comment if you have any questions.
Resources:
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings