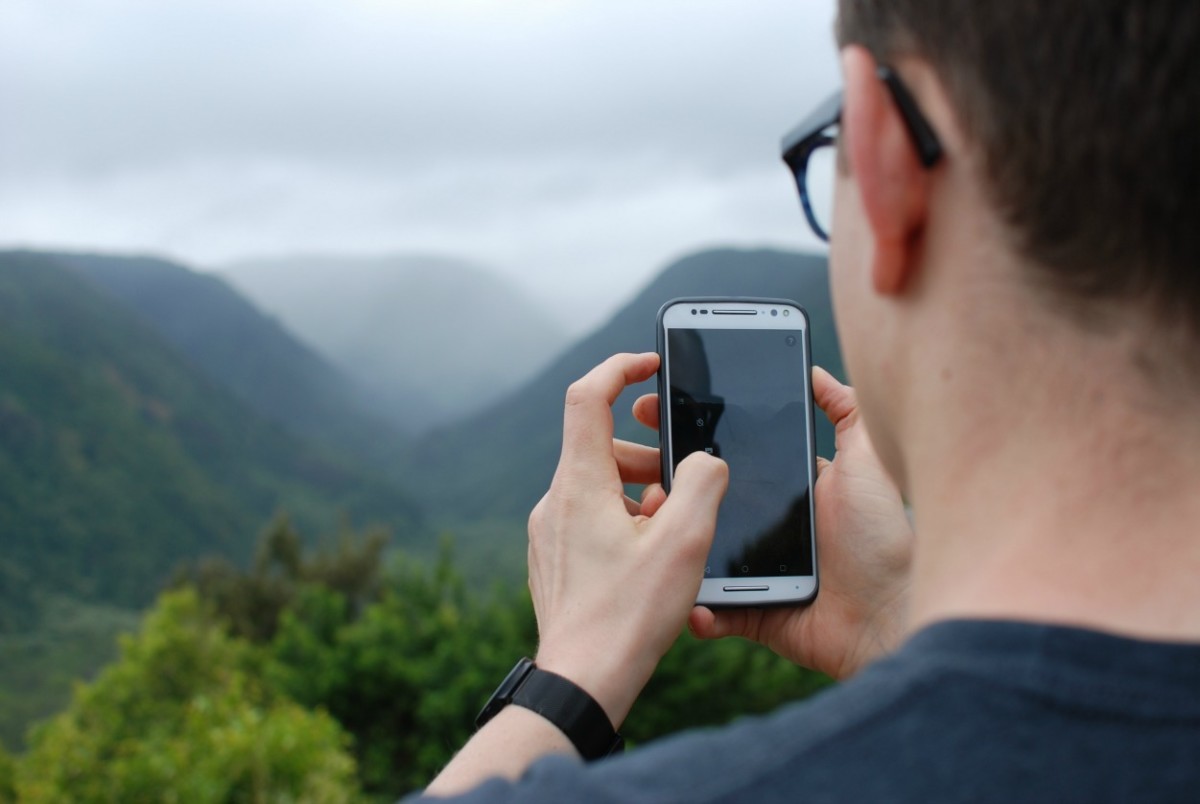
Beginning development for a new platform can be a difficult process—and even more so without the helpful libraries that people have already created to ease the process. “Reinventing the wheel” in software development will slow development time and weaken your code stability. You will have more code to maintain and more opportunities for bugs and errors to pop up. There are many libraries for Android that will make your life easier. We attended an Android BBQ talk by Chris Guzman that outlined a few Android libraries that are great to know if you are just getting started:
GSON
GSON can easily turn Java classes into JSON objects and vice versa. If you have spent five minutes working with JSON objects without a competent JSON parsing library then you are already familiar with the tedious and frustrating process of creating a custom parser yourself. By using the class variable names, GSON automatically serializes a JAVA object into a JSON object using the variable names as property names in the object. If you want more control over the objects, you can customize names and properties using annotations in your JAVA objects. It’s clean, fast, and requires less development than other parsers.
There are several other great JSON parsing libraries for JAVA, and in many cases, choosing between them comes down to preference. GSON is easy to learn, and the code is easy to understand, making it a solid choice for those just getting started with Android development.
RETROFIT
Retrofit is a type-safe HTTP client that is tailored to work with standard REST APIs. To create a service layer in Retrofit you can define a Java interface with your API methods and use the Retrofit annotations to define how you want to make that request. This is best illustrated in the following code, which was pulled from the Retrofit website.
public interface GitHubService {
@GET("users/{user}/repos")
Call<List<Repo>> listRepos(@Path("user") String user);
}
That’s all that is involved in defining your API interface. You can pass this interface into Retrofit’s create method and it will give you back a service to make your HTTP requests with. There is even a provided integration with the previously mentioned GSON library. With these two libraries your application’s service layer will be easy to maintain.
REALM
Unless you want to make a network call every few seconds, it is very likely that your app will require some data persistence on the user’s device. SQLite has been the answer to this problem for a long time for Android development, but Realm has recently gained a lot of popularity. The two main reasons for this are ease of use and performance. Realm is extremely easy to implement and has been shown to outperform the competition on mobile apps.
Realm also comes with a handy utility called the “Realm Browser” that allows you to view and edit the realm database on a device. This is a great tool for development and debugging your code. On top of all of this, Realm is platform-agnostic, meaning that you can share the same database files between your Android and iOS development.
Oh, and while there is a premium version of the platform that you can purchase, the base version includes everything mentioned above, and it is completely free. There are a lot of developers who are accustomed to using SQLite and other database tools, but with all that Realm has to offer you should definitely consider it for your mobile app.
PICASSO
Retrieving remote images and displaying them in an ImageView is a very common use case for any mobile application. The Android code to do this can be very verbose and can contain a lot of “boilerplate” to handle errors and decode the image into the proper format. Picasso makes this easier and handles the download, caching, and manipulation of remote images for you. The following example allows us to retrieve the image, cache it, resize it, crop it, and finally place it into the desired image view in one line.
Picasso.with(context)
.load(url)
.resize(50, 50)
.centerCrop()
.into(imageView)
Compared to the pure Android approach, this code is easier to read and maintain. In addition, Picasso also provides methods to introduce image placeholders, custom image transformations, error indicators for when an image fails to load, and loading indicators to inform the user when an image is currently being loaded for a view. This all comes free to you with a single function call from the Picasso class, and you don’t have to write a single line of code.
CONCLUSION
These four great libraries can go a long way in making your code more clear, concise, and maintainable. They can also reduce the barrier to entry if this is your first Android application. Let’s keep ourselves from “reinventing the wheel” in our applications and utilize these open source projects. Let us know in the comment section if you have used any of these libraries and how they impacted your experience.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings