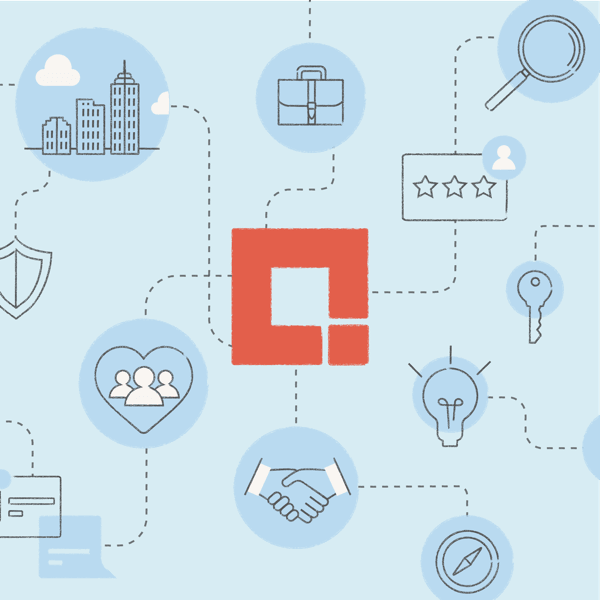
I’ve been working with Microsoft Dynamics CRM for more than four years, and during that time I have done just about every type of customization and enhancement you can think of to personalize CRM for my clients. As new versions have been released and new features made available, I have always been eager to try them out and see what new flexibility and capability can be achieved. However, throughout all of that, there is one particular type of customization that I have never had the need to utilize until now. My current client had some unique requirements for workflows, which led me to research and ultimately develop several new custom workflow activities. Custom workflow activities fall somewhere between workflows and plugins in terms of level of difficulty to implement, but they can be very powerful and provide a great tool to fill the gaps in standard workflow activities.
For those of you who are new to CRM or just new to custom workflow activities, let me take a moment to explain them. Custom workflow activities allow you to add different types of “steps” to the existing “Add Step” menu in the workflow designer. For example, one of the options available to you in the “Add Step” menu currently on the workflow is “Send Email. By adding this step, you can create and send a dynamic email activity populated with data from the record which triggered the workflow. Custom workflow activities allow you to add additional functionality as steps of the workflow in the same way.
– Have you ever needed to embed a link to a record inside the body of an email using a workflow?
– Have you ever needed to format a DateTime property so it only showed the Date and not the Time?
– Have you ever needed to add, subtract, multiply, or divide two numbers in order to create a calculated field?
If so, you are in luck. In this post, I’m going to explain how you would use custom workflow activities to add this functionality to your workflows.
GETTING STARTED
If you are using the CRM Developer Toolkit for Visual Studio, the process is very straightforward. Simply create a CRM Workflow project in Visual Studio and then add a “Custom Workflow Activity” item to your project. If not, you can find many good examples in the CRM 2013 SDK (SDK\SampleCode\CS\Process\CustomWorkflowActivities). Once you have a new “CodeActivity” class created, you need to add a few things to customize the activity.
GENERATE A LINK TO A CRM RECORD
The “regarding” field in an email generated from CRM is great, but it is only great if your recipient is using the CRM Outlook client. Even then, it is not necessarily easy to find. You may also have the situation where you want to link to multiple different records from within an email message. This can be accomplished using a Custom Workflow Activity which will dynamically generate a link that can be placed in the body of a workflow generated email.
Inside your new Custom Workflow Activity class, you will first need to add a few parameters. When you add this custom activity to a workflow in CRM, these parameters are the fields which are populated to tell the activity which record you want the hyperlink to point to. For this example I am going to create an activity which will generate a link to a Campaign record. I will create four parameters:
The first will capture the CRM URL prefix.
The second will capture the particular Campaign record I want to link to.
I will create an optional parameter to capture the friendly text that I want to display in the hyperlink.
The fourth is the output parameter that sends the resulting hyperlink text back to the workflow.
// Define Input/Output Arguments
[Input(“Campaign Link Text”)]
public InArgument<string> campaignLinkText { get; set; }
[RequiredArgument]
[Input(“CRM URL”)]
[Default(“https://credera2.crm.dynamics.com/”)]
public InArgument<string> crmURL { get; set; }
[RequiredArgument]
[Input(“Campaign”)]
[ReferenceTarget(“campaign”)]
public InArgument<EntityReference> inputCampaign { get; set; }
[Output(“Campaign URL”)]
public OutArgument<string> campaignURL { get; set; }
Of course, you could make a CRM service call to get the CRM URL prefix but for the purposes of this example, I am going to ask the user to enter it. This is an important step, especially if you are using an on-premise version of CRM and have multiple environments such as development and QA. The code for the parameters will look something like this:
try
{
string hyperlink = string.Empty;
string url = string.Concat(
this.crmURL,
“ma/camps/edit.aspx?id={“,
this.inputCampaign.Get(executionContext).Id.ToString(),
“}#”);
if (string.IsNullOrEmpty(this.campaignLinkText.Get(executionContext)))
hyperlink = “<a href=” + url + “>” + url + “</a>”;
else
hyperlink = “<a href=” + url + “>” + this.campaignLinkText.Get(executionContext) + “</a>”;
this.campaignURL.Set(executionContext, url);
}
catch (FaultException<OrganizationServiceFault> e)
{
tracingService.Trace(“Exception: {0}”, e.ToString());
throw;
}
Once the parameters are defined and captured, you must then implement the CodeActivity.Execute method to generate the hyperlink based on the input parameters. Once again, if you are using the CRM Development Toolkit, your auto-generated custom workflow activity class will have the Execute method defined and filled with helper code to get you started. You just need to retrieve the parameter values, generate the hyperlink, and then populate the output parameter. I am using the string concatenate function to join the CRM URL, with the Campaign ID. Then, if a value has been provided for Campaign Text, I use that to give a friendly name to my hyperlink.
DATETIME FORMATTING
Custom workflow activities can also come in handy for text formatting. In this example I want to display a date in the subject line and body of an email, but I do not want to see the “12:00:00” which will show up after the date. I also want a little more flexibility and options on how that date is formatted. I may want to print it out as “5/20/2014” in one part of the email, but show it as “Tuesday, May 20th 2014” in another part of the email.
First, we need to create our input and output parameters. The first input will be the CRM date that we want to display, and the second parameter will be the date format string, which will dictate how the date is formatted. We are going to assume our workflow creator has some C# experience and understands how the date format string works and what the options are for formatting dates.
// Define Input/Output Arguments
[RequiredArgument]
[Input(“Date Format String”)]
public InArgument<string> formatString { get; set; }
[RequiredArgument]
[Input(“Date to Format”)]
public InArgument<DateTime> dateToFormat { get; set; }
[Output(“Formatted Date”)]
public OutArgument<string> formattedDate { get; set; }
Once you have the parameters created, implementing the Execute method is a simple task of checking the format string for null (always check for null, no matter how confident you are that it could not possibly be null) and applying it to the DateTime value. In this example if for some reason the format string is null, then I am just returning the standard result from the DateTime.ToString() function.
string format = formatString.Get(executionContext);
DateTime date = dateToFormat.Get(executionContext);
if (!string.IsNullOrEmpty(format))
formattedDate.Set(executionContext, date.ToString(format));
else
formattedDate.Set(executionContext, date.ToString());
BASIC MATH
I won’t go into detail, but another example of where this might come in handy is for performing simple math calculations. Say you want to count the number of Contacts associated to an Account and store that on the Account record. Or you may need to add two numbers together and then divide to calculate a percentage. Many of the out-of-the-box entities in CRM have the magical ability to rollup cost calculations from child entities to the parent, such as Opportunities and Quotes. You could create custom workflow activities and the Workflows to handle these calculations much in the same way I have shown above.
Now that we have our custom workflow activities coded, we need to upload them to CRM using the plugin registration tool and then use them in our workflows. When you click on the “Add Step” button, you will now see a new grouping at the bottom of the menu. This grouping will contain your new custom workflow activities
When you select to add the “Create Campaign Link” activity, you will be presented with a screen to enter in the parameters that you set up in the code. When it comes time to identify the Campaign object to link to, use the Form Assistant to select the Campaign object and add it to the step input properties using the “Add” button
Lastly, create a new step to “Send Email.” Inside the “Set Properties” of that email, you can access the generated campaign URL using the Form Assistant and look for the name you gave the “Create Campaign Link” step. Selecting that from the “Look for:” picklist should then populate the next picklist with your generated Campaign URL. This value represents the Output parameter you defined in your custom workflow activity class. Save and close this workflow and create a campaign to see what happens next!
Credera has extensive experience in deploying and customizing Dynamics CRM for our clients. We are always looking for opportunities to share our experiences and help companies through the difficult business and technical decisions that come with implementing a CRM product. Please contact us on Twitter @CrederaMSFT or leave a comment below for more information.
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings