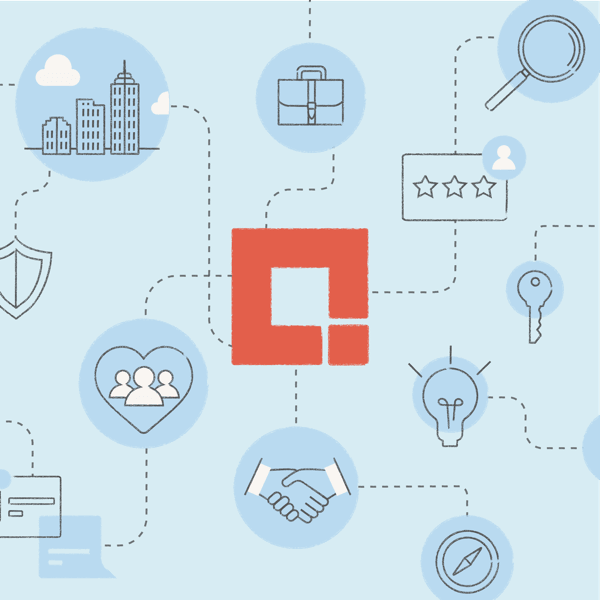
This is the second part of my series describing how I have learned to more easily test my AngularJS projects. In part one, Setting up Unit Testing With Karma, we configured Karma to run our unit tests. In this post, I will talk about how you can use three additional plugins to extend Karma and enhance your testing.
Be sure to check out the example app for a full, working implementation.
Include HTML Files With karma-ng-html2js-preprocessor
For unit testing directives that use the templateUrl setting or for unit testing routes, you can install an additional Karma plugin to convert HTML files to JS files. Otherwise, running the tests might throw this error: “Unexpected request: GET partials/directive.html No more request expected”.
Install the plugin with Node.
npm install karma-ng-html2js-preprocessor --save-dev
Update the karma-conf.js file. See the example project on GitHub for the full configuration file.
// add html files to the list of included files files: [ 'app/partials/directives/*.html', 'app/partials/*.html', ... ]
// add html files to list of files for preprocessing preprocessors: { 'app/partials/directives/*.html': 'html2js', 'app/partials/*.html': 'html2js' },
// add to the list of plugins plugins : [ 'karma-ng-html2js-preprocessor', ... ],
// add the plugin settings ngHtml2JsPreprocessor: { stripPrefix: 'app/' }
Now you can include HTML files as modules in unit test specs and no errors should be thrown.
beforeEach(module("partials/directives/directiveTemplate.html"));
Test Coverage With karma-coverage
Test coverage is a measurement that describes how much of you code has been tested. The karma-coverage plugin will report this so you know how much of your app is tested with your unit tests.
Install the coverage plugin with Node.
npm install karma-coverage --save-dev
Configure the plugin settings in the karma-conf.js file.
// add to list of reporters reporters: ['progress', 'coverage'],
// add to list of plugins plugins: [ 'karma-coverage', ... ],
// add to list of preprocessors preprocessors: { 'app/js/controllers/*.js': ['coverage'], 'app/js/directives/*.js': ['coverage'], 'app/js/app.js': ['coverage'] },
// add plugin settings coverageReporter: { // type of file to output, use text to output to console type : 'text', // directory where coverage results are saved dir: 'test-results/coverage/' // if type is text or text-summary, you can set the file name // file: 'coverage.txt' }
Now run the karma start test/karma-conf.js –single-run command. You should see your coverage results when the tests are done running.
JSHint Messages With karma-jshint-preprocessor
JSHint is a tool that will detect errors and violations of best practices in JavaScript. You can install a Karma plugin to run JSHint as part of your testing process.
Install the plugin with Node.
npm install karma-jshint-preprocessor --save-dev
Configure the plugin settings in the karma-conf.js file.
// add to list of plugins plugins: [ 'karma-jshint-preprocessor', ... ],
// add to list of preprocesssors preprocessors: { 'app/js/controllers/*.js': ['jshint', 'coverage'], 'app/js/directives/*.js': ['jshint', 'coverage'], 'app/js/app.js': ['jshint', 'coverage'] },
// add plugin settings junitReporter: { // location of results output file outputFile: 'test-results/junit-results.xml' }
You can configure your JSHint options in a .jshintrc file located at the root of your project. The file in the example project looks as follows. JSHint was designed to be configurable, so there are several other options available.
{ // suppresses warnings about the use of global strict mode "globalstrict": "false", // define globals "predef": ["angular"] }
Now run the karma start test/karma-conf.js –single-run command. You should see a list of JSHint errors if you have any.
Conclusion
Karma has several useful plugins that can enhance your testing process. Be sure to check if there is one you could use or create your own. In the next post, I will explain how to set up Karma and Grunt, so you can integrate Karma with your build process.
Sources
Contact Us
Ready to achieve your vision? We're here to help.
We'd love to start a conversation. Fill out the form and we'll connect you with the right person.
Searching for a new career?
View job openings